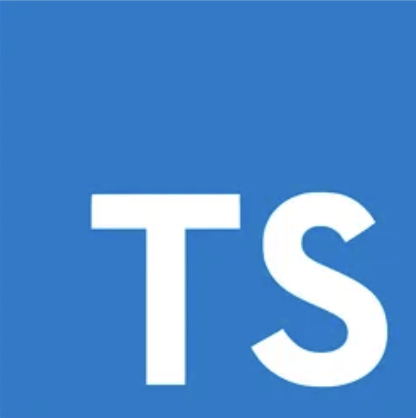
In TypeScript, when rendering a number directly within JSX, you may encounter the error “Type ‘Number’ is not assignable to type ‘ReactNode’.” This error occurs because JSX expects elements or strings, not primitive types like numbers, to be rendered directly.
To render a number within JSX without encountering this error, you can convert the number to a string using the toString()
method or template literals. Here’s how you can do it:
const myNumber: number = 42;
// Using toString() method
const jsxElement1 = <div>{myNumber.toString()}</div>;
// Using template literals
const jsxElement2 = <div>{`${myNumber}`}</div>;
Both of these approaches convert the number 42 to a string “42”, which JSX can render without any issues.
Alternatively, you can use the String() function to convert the number to a string:
const jsxElement3 = <div>{String(myNumber)}</div>;
All of these methods will allow you to render a number within JSX without encountering the “Type ‘Number’ is not assignable to type ‘ReactNode’” error. Choose the method that best fits your coding style and preferences.
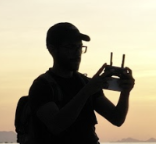
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply