State management is a core concept in ReactJS, essential for building dynamic and interactive web applications. In intercation between customer/user to a web application there’s a lot of data that flow between the user to what the user see’s and what exists in the database and in the backend of our system. In some cases that data belong to the database and needs to be save and in some cases that data is relevant to what the user see. As a professional software engineer, I have extensive experience in managing state in React applications, which has allowed me to understand its intricacies and best practices. In this article, I’ll share insights from my personal experience on how to effectively handle state in ReactJS.
What is State in React?
In ReactJS, state refers to the data that changes over time and influences what gets rendered on the user interface (UI). Unlike props, which are read-only and passed down from parent to child components, state is local to the component and can be changed using the setState
function or the useState
hook in functional components. State allow our UI to be interactive, dynamic, change base on the needs of our product. State improve UX and the performance of our application by performing actions on-the-surface rather than re-rendering all our application or notifying the backend with API calls every time we perform any action.
Class Components and State
In class components, state is an object that is initialized in the constructor and managed using the setState
method.
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
increment = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.increment}>Increment</button>
</div>
);
}
}
export default Counter;
Functional Components and Hooks
With the introduction of hooks in React 16.8, functional components can also manage state using the useState hook.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
State Initialization and Updates
State can be initialized with any value, including objects, arrays, or even functions. When updating state, it’s important to remember that React batches state updates for performance optimization. Therefore, updates are asynchronous.
const [user, setUser] = useState({ name: '', age: 0 });
const updateName = (newName) => {
setUser((prevUser) => ({ ...prevUser, name: newName }));
};
In this example, the updateName function correctly updates the name property while preserving the other properties of the user object.
Best Practices for Managing State
Keep State Local Where Possible: Only lift state up to the nearest common ancestor if multiple components need access to it.
Use Functional Updates: When the new state depends on the previous state, use functional updates to avoid bugs due to asynchronous updates.
setCount((prevCount) => prevCount + 1);
Avoid Deeply Nested State: Deeply nested state can lead to performance issues and complex state management. Flatten state where possible.
Leverage Context for Global State: For global state management, such as user authentication or theme settings, use React Context or state management libraries like Redux or Zustand.
const UserContext = React.createContext();
Memoize Expensive Calculations: Use useMemo and useCallback hooks to memoize expensive calculations or functions that depend on state.
const memoizedValue = useMemo(() => computeExpensiveValue(state), [state]);
Handle Side Effects Properly: Use the useEffect hook to handle side effects, such as data fetching or subscriptions, which depend on state.
useEffect(() => {
fetchData();
}, [state]);
State Management Libraries
For complex applications, managing state solely with React’s built-in capabilities can become cumbersome. State management libraries like Redux, MobX, or Recoil provide more powerful and scalable solutions.
Example with Redux:
Setup Redux Store:
import { createStore } from 'redux';
const store = createStore(reducer);
Connect Component to Redux:
import { useSelector, useDispatch } from 'react-redux';
function Counter() {
const count = useSelector((state) => state.count);
const dispatch = useDispatch();
const increment = () => {
dispatch({ type: 'INCREMENT' });
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
Conclusion
State management is crucial for developing dynamic and interactive React applications. Understanding the differences between class and functional components, using hooks effectively, and following best practices for state management can greatly enhance your React development experience. For more complex scenarios, consider using state management libraries to keep your application organized and maintainable. By mastering these concepts, you’ll be well-equipped to build robust and efficient React applications.
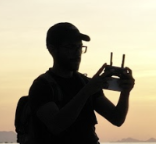
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply