In React, you can use the useEffect hook with an empty dependency array [] to execute the effect only once when the component mounts. Here’s how you can do it:
import React, { useEffect } from 'react';
const YourComponent: React.FC = () => {
useEffect(() => {
// Your logic here
console.log('Component mounted');
// Clean up the effect (optional)
return () => {
console.log('Component unmounted');
};
}, []); // Empty dependency array
// Your component JSX
return (
<div>
{/* Your component content */}
</div>
);
};
export default YourComponent;
In the above example:
- We pass an empty dependency array
[]
as the second argument to theuseEffect
hook. - This tells React that the effect doesn’t depend on any values from the component’s props or state, so it should only run once when the component mounts.
- Inside the effect function, you can perform any initialization logic or side effects that you want to execute when the component mounts.
- Optionally, you can return a cleanup function from the effect to perform any necessary cleanup when the component unmounts.
Examples – One Time Execute
Fetch Data
When we want to fetch data once we can use the useEffect with empty array dependencies to execute once. Let’s take a look at the code example
import React, { useEffect, useState } from 'react';
const MyComponent: React.FC = () => {
const [data, setData] = useState(null);
useEffect(() => {
console.log('Component mounted');
// Fetch data from API
fetchData().then((response) => setData(response.data));
}, []);
return (
<div>
{/* Component content */}
</div>
);
};
export default MyComponent;
Setting Up Event Listeners
You can use useEffect to add event listeners when the component mounts and remove them when the component unmounts. This ensures that the event listeners are set up only once.
import React, { useEffect } from 'react';
const MyComponent: React.FC = () => {
useEffect(() => {
const handleClick = () => {
console.log('Button clicked');
};
window.addEventListener('click', handleClick);
return () => {
window.removeEventListener('click', handleClick);
};
}, []); // Empty dependency array ensures one-time execution
return (
<div>
{/* Component content */}
</div>
);
};
export default MyComponent;
Performing Cleanup Tasks
You can use useEffect with a cleanup function to perform cleanup tasks, such as clearing timeouts or intervals, when the component unmounts. This ensures that resources are properly cleaned up after the component is removed from the DOM.
import React, { useEffect } from 'react';
const MyComponent: React.FC = () => {
useEffect(() => {
const timerId = setTimeout(() => {
console.log('Timer expired');
}, 1000);
return () => {
clearTimeout(timerId);
};
}, []); // Empty dependency array ensures one-time execution
return (
<div>
{/* Component content */}
</div>
);
};
export default MyComponent;
Initializing Third-Party Libraries
You can use useEffect to initialize third-party libraries or perform other setup tasks that only need to happen once when the component mounts.
import React, { useEffect } from 'react';
import { Chart } from 'chart.js';
const MyComponent: React.FC = () => {
useEffect(() => {
const chart = new Chart('myChart', {
type: 'bar',
data: {
labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'],
datasets: [{
label: '# of Votes',
data: [12, 19, 3, 5, 2, 3],
backgroundColor: [
'rgba(255, 99, 132, 0.2)',
'rgba(54, 162, 235, 0.2)',
'rgba(255, 206, 86, 0.2)',
'rgba(75, 192, 192, 0.2)',
'rgba(153, 102, 255, 0.2)',
'rgba(255, 159, 64, 0.2)'
],
borderColor: [
'rgba(255, 99, 132, 1)',
'rgba(54, 162, 235, 1)',
'rgba(255, 206, 86, 1)',
'rgba(75, 192, 192, 1)',
'rgba(153, 102, 255, 1)',
'rgba(255, 159, 64, 1)'
],
borderWidth: 1
}]
},
options: {
scales: {
y: {
beginAtZero: true
}
}
}
});
return () => {
// Cleanup chart instance if needed
};
}, []); // Empty dependency array ensures one-time execution
return (
<div>
<canvas id="myChart" width="400" height="400"></canvas>
</div>
);
};
export default MyComponent;
These examples demonstrate how useEffect can be used to achieve one-time execution behavior similar to a constructor in functional components. The more you’ll dive into your software engineering skills and product requests you’ll find more usecases for those one-time life-cycle react components usecases. Over time you’ll distinguish between the two more.
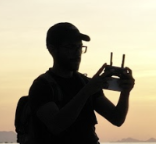
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply