In React.js, props (short for properties) are a way to pass data from a parent component to a child component. Props are read-only, meaning that the child component cannot modify the props it receives from its parent. Props are a fundamental concept in React.js and are used to customize and configure components.
Using Props in Functional Components
In a functional component, props are passed as an argument to the component function. Here’s an example:
import React from 'react';
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
const App = () => {
return <Greeting name="Alice" />;
};
export default App;
In the above jsx example, the ‘Greeting’ component receives a ‘name’ prop from its parent ‘App’ component. The ‘Greeting’ component then uses this prop to display a personalized greeting.
Default Props
You can also specify default values for props using the ‘defaultProps’ property. Here’s an example:
import React from 'react';
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
Greeting.defaultProps = {
name: 'Guest'
};
const App = () => {
return <Greeting />;
};
export default App;
In this example, if the ‘name’ prop is not provided to the ‘Greeting’ component, it will default to ‘Guest’.
Passing Functions as Props
Props can also be used to pass functions from a parent component to a child component. This allows the child component to communicate with the parent component. Here’s an example:
import React from 'react';
const Button = (props) => {
return <button onClick={props.onClick}>Click me</button>;
};
const App = () => {
const handleClick = () => {
alert('Button clicked!');
};
return <Button onClick={handleClick} />;
};
export default App;
In this example, the App component defines a handleClick function, which is passed to the Button component as a prop. When the button is clicked, the handleClick function is called, showing an alert.
Pros And Cons of Props
Pros | Cons |
---|---|
Reusable Components: Props allow you to create reusable components by passing different data to them. | Prop Drilling: In complex component trees, passing props through multiple levels can become cumbersome, leading to prop drilling. |
Component Customization: Props enable you to customize the behavior and appearance of components based on the data passed to them. | Immutable Props: Props are immutable, meaning they cannot be changed by the component receiving them, which can lead to extra complexity when managing state. |
Component Composition: Props facilitate component composition, allowing you to build complex UIs from simple, reusable components. | Type Safety: In JavaScript, props are not type-safe by default, which can lead to runtime errors if not handled carefully. |
Easy Data Flow: Props provide a simple and predictable way to manage data flow in your application, making it easier to debug and maintain. | Performance Overhead: Passing props to components can have a performance cost, especially in large applications with many components. |
Separation of Concerns: Props promote separation of concerns by keeping the data management logic separate from the presentation logic. | Complex Component Interfaces: Using props extensively can lead to components with large and complex interfaces, making them harder to understand and maintain. |
Conclusion
Props are a powerful feature in React.js that allow you to pass data and functions between components. They enable you to create reusable and customizable components, making your React applications more dynamic and interactive.
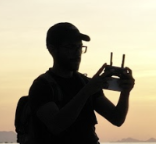
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply