Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It’s a way of writing software that emphasizes functions, immutability, and declarative programming. In this article, we’ll explore the key concepts of functional programming and compare it with object-oriented programming.
Key Concepts of Functional Programming
Pure Functions
Pure functions are functions that always return the same result for the same input and have no side effects. They depend only on their input arguments and do not modify the state of variables outside the function. For example:
function add(a, b) {
return a + b;
}
Immutability
Immutability is the principle that once a data structure is created, it cannot be changed. Instead, new data structures are created when modifications are needed. This prevents unintended side effects and makes code easier to reason about.
Higher-Order Function
Higher-order functions are functions that can take other functions as arguments or return functions as results. This allows for the creation of more complex and reusable code.
Function Composition
Function composition is the act of combining two or more functions to produce a new function. This allows for the creation of complex behaviors by chaining together simpler functions.
Functional Programming & Object-Oriented Programming
In object-oriented programming (OOP), the emphasis is on objects and their interactions, while in functional programming (FP), the emphasis is on functions and their transformations of data.
In OOP, objects have state and behavior, and methods can modify the state of an object. In FP, data is immutable, and functions are pure, meaning they do not have side effects and do not modify state.
Comparing Functional Programing to Object Oriented
Aspect | Functional Programming | Object-Oriented Programming |
---|---|---|
Basic Concept | Focuses on functions as the primary building blocks | Focuses on objects as the primary building blocks |
State Management | Emphasizes immutability and avoids mutable state | Uses objects with mutable state |
Data Transformation | Emphasizes transformations through function calls | Emphasizes operations on objects using methods |
Control Flow | Emphasizes recursion and higher-order functions | Emphasizes loops and method calls for control flow |
Inheritance | Uses composition and higher-order functions | Uses class-based inheritance and polymorphism |
Code Reusability | Achieved through higher-order functions and composition | Achieved through class hierarchies and inheritance |
Side Effects | Discourages side effects and encourages pure functions | Allows side effects through object state modification |
Concurrency | Promotes immutability for easier concurrency | Often requires explicit synchronization for concurrency |
Learning Curve | May have a steep learning curve for beginners | Often more intuitive for beginners due to real-world analogy |
Examples | Languages like Haskell, Lisp, and Erlang | Languages like Java, C++, and Python |
Benefits of Functional Programming
- Easier Testing: Pure functions are easier to test because they always produce the same output for the same input.
- Concurrency: Functional programming encourages immutability, which makes it easier to write concurrent programs.
- Code Reusability: Higher-order functions and function composition promote code reuse and modularity.
Code Examples
Here are two examples, one demonstrating functional programming and the other object-oriented programming, both accomplishing the same task of calculating the sum of an array of numbers.
Functional Programming Example:
// Functional Programming
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // Output: 15
Object-Oriented Programming Example:
// Object-Oriented Programming
class SumCalculator {
constructor(numbers) {
this.numbers = numbers;
}
calculateSum() {
return this.numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
}
}
const numbers = [1, 2, 3, 4, 5];
const sumCalculator = new SumCalculator(numbers);
const sum = sumCalculator.calculateSum();
console.log(sum); // Output: 15
In the functional programming example, the reduce method is used to iterate over the array and calculate the sum. In the object-oriented programming example, a class SumCalculator is defined with a method calculateSum to calculate the sum of the array numbers.
Functional Programming Example
Let’s take a look at another example from a real world case of function programing for a basic react’ components, the recommended approach by Meta(facebook) which stands behind ReactJS
// Functional Programming
import React from 'react';
const ItemList = ({ items }) => (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
export default ItemList;
Object-Oriented Programming Example
// Object-Oriented Programming
import React, { Component } from 'react';
class ItemList extends Component {
render() {
return (
<ul>
{this.props.items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
}
export default ItemList;
In the functional programming example, a functional component ItemList
is defined using an arrow function. It takes a prop items
and uses the map
method to render a list of items.
In the object-oriented programming example, a class ItemList
is defined that extends Component
. It defines a render
method that achieves the same functionality as the functional component.
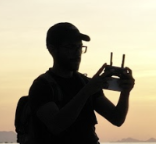
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply