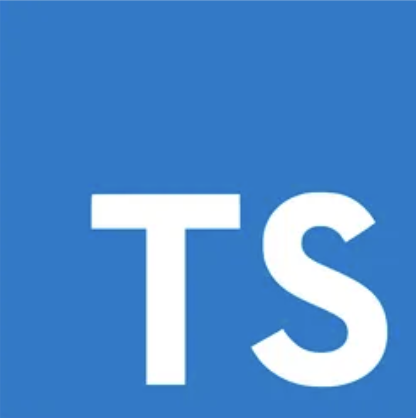
In TypeScript, you can define createdAt
and updatedAt
fields in an interface to represent timestamps using either the Date
type or a custom type. Here’s how you can define them using the Date
type:
interface MyInterface {
// Other fields...
createdAt: Date;
updatedAt: Date;
}
This approach uses the built-in Date
object provided by JavaScript. Alternatively, if you want to use a custom type for timestamps, you can define it like this:
type Timestamp = number;
interface MyInterface {
// Other fields...
createdAt: Timestamp;
updatedAt: Timestamp;
}
In this example, we define a Timestamp
type as a number
, which represents the number of milliseconds since the Unix epoch. You can customize this type according to your requirements, such as using a string
type to represent ISO 8601 formatted timestamps.
Choose the approach that best fits your use case and coding style. Both approaches are valid, and the choice depends on your specific needs and preferences.
I always remember that typescript help us with many things which why pushing and trying more eventually helps. Typescript provide us many advantages such as Static Typing, TypeScript introduces static typing to JavaScript, allowing developers to define explicit types for variables, parameters, and return values. This helps catch type-related errors during development, leading to fewer runtime errors and improved code reliability. Enhanced Code Readability, With type annotations, TypeScript code becomes more self-documenting, making it easier for developers to understand the purpose and expected usage of functions, classes, and variables. This can improve code maintainability, especially in large codebases with multiple contributors.
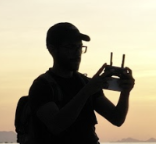
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply