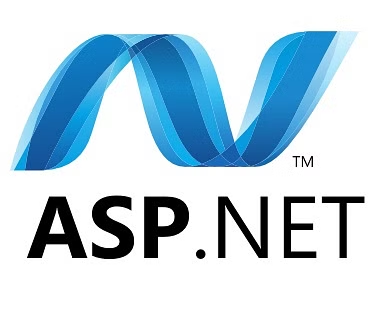
“Razor” dot files, also known as “.cshtml” files, are associated with the ASP.NET Razor syntax, which is a markup syntax used to create dynamic web pages in ASP.NET applications. These files contain a mixture of HTML and C# or VB.NET code that is processed on the server to generate the final HTML output sent to the client’s web browser.
The Razor syntax provides a concise and expressive way to embed server-side code within HTML markup, making it easier for developers to create dynamic and data-driven web pages. In Razor files, server-side code is typically enclosed within special delimiters (@
symbol) and can be used to execute logic, access data from the server, and generate dynamic content.
Here’s a simple example of a Razor file:
<!DOCTYPE html>
<html>
<head>
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome, @Model.Name!</h1>
<p>Your account balance is: $@Model.Balance</p>
</body>
</html>
In this example, @Model.Name
and @Model.Balance
are expressions that retrieve dynamic data from the server-side code and embed it within the HTML markup. When the Razor file is processed on the server, these expressions are replaced with the actual data values before being sent to the client’s browser.
Overall, Razor dot files are a crucial part of ASP.NET web development, allowing developers to create dynamic and interactive web applications with ease.
PHP Blade Templating Example
examples of how dynamic data retrieval is done in Laravel (a PHP framework) and in Node.js (using a popular templating engine called EJS). Let’s assume we want to achieve the same functionality as the Razor example provided earlier, where we display the user’s name and account balance on a web page.
Laravel Example (Blade Templating Engine)
Assuming we have a route set up to render the view, and we pass the user’s name and account balance to the view from the controller:
// Controller logic to retrieve user data
$user = Auth::user(); // Assuming the user is authenticated
$name = $user->name;
$balance = $user->balance;
// Pass data to the view
return view('welcome', compact('name', 'balance'));
Now, in the welcome.blade.php
file (equivalent to a Razor file in Laravel), we can access these variables directly:
<!DOCTYPE html>
<html>
<head>
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome, {{ $name }}!</h1>
<p>Your account balance is: ${{ $balance }}</p>
</body>
</html>
Nodejs EJS Templating – Example
Node.js (with EJS Templating Engine) Example: First, make sure you’ve set up Express.js with EJS as the view engine:
const express = require('express');
const app = express();
app.set('view engine', 'ejs'); // Set EJS as the view engine
// Route to render the view
app.get('/', (req, res) => {
const user = { name: 'John Doe', balance: 1000 }; // Example user data
res.render('welcome', { user });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Now, in the welcome.ejs file (equivalent to a Razor file in Node.js with EJS), we can access the user object properties:
<!DOCTYPE html>
<html>
<head>
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome, <%= user.name %>!</h1>
<p>Your account balance is: $<%= user.balance %></p>
</body>
</html>
In both examples, we are retrieving dynamic data (user’s name and account balance) from the server-side code (controller in Laravel, route handler in Express.js) and embedding it within the HTML markup using the Blade templating engine in Laravel and the EJS templating engine in Node.js.
Differences Between Razor
Let’s take a look a bit of the difference between Razor templating to nodejs ejs and blade templating of php/laravel framework.
Feature | Blade Templating (PHP/Laravel) | Node.js with EJS | Razor (ASP.NET) |
---|---|---|---|
File Extension | .blade.php | .ejs | .cshtml |
Syntax for Variables | {{ $variable }} | <%= variable %> | @variable |
Conditional Statements | @if(condition) { ... } @endif | <% if(condition) { %> <% } %> | @if (condition) { ... } |
Looping Constructs | @foreach($items as $item) { ... } @endforeach | <% items.forEach(function(item) { %> <% }); %> | @foreach(var item in items) { ... } |
Layouts/Inheritance | Supported | Supported | Supported |
Partials/Includes | @include('partial.view') | <%- include('partial.ejs') %> | @Html.Partial("_PartialView") |
Comments | {{-- Comment --}} | <%# Comment %> | @* Comment *@ |
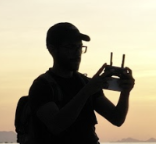
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply